1. (a) What is Object Oriented Programming? Explain concepts of object and
class, with the help of example of each.
A.1(a)
(b) What is information hiding? Explain its advantages.
A.1.(b)
A.1.(c)
A.1(a)
Object Oriented programming is a programming style that is associated with the concept of Class, Objects and various other concepts revovling around these two, like Inheritance, Polymorphism, Abstraction, Encapsulation etc.
Let us try to understand a little about all these, through a simple example. Human Beings are living forms, broadly categorized into two types, Male and Female. Right? Its true. Every Human being(Male or Female) has two legs, two hands, two eyes, one nose, one heart etc. There are body parts that are common for Male and Female, but then there are some specific body parts, present in a Male which are not present in a Female, and some body parts present in Female but not in Males.
All Human Beings walk, eat, see, talk, hear etc. Now again, both Male and Female, performs some common functions, but there are some specifics to both, which is not valid for the other. For example : A Female can give birth, while a Male cannot, so this is only for the Female.
Human Anatomy is interesting, isn't it? But let's see how all this is related to C++ and OOPS. Here we will try to explain all the OOPS concepts through this example and later we will have the technical definitons for all this.
- Object − Objects have states and behaviors. Example: A dog has states - color, name, breed as well as behaviors – wagging the tail, barking, eating. An object is an instance of a class.
- Class − A class can be defined as a template/blueprint that describes the behavior/state that the object of its type support.
Objects in Java
Let us now look deep into what are objects. If we consider the real-world, we can find many objects around us, cars, dogs, humans, etc. All these objects have a state and a behavior.
If we consider a dog, then its state is - name, breed, color, and the behavior is - barking, wagging the tail, running.
If you compare the software object with a real-world object, they have very similar characteristics.
Software objects also have a state and a behavior. A software object's state is stored in fields and behavior is shown via methods.
So in software development, methods operate on the internal state of an object and the object-to-object communication is done via methods.
Classes in Java
A class is a blueprint from which individual objects are created.
Following is a sample of a class.
Example
public class Dog
{
String breed;
int age;
String color;
void barking()
{
}
void hungry()
{
}
void sleeping()
{
}
}
(b) What is information hiding? Explain its advantages.
A.1.(b)
Information hiding is one of the most important principles of OOP inspired from real life which says that all information should not be accessible to all persons. Private information should only be accessible to its owner.
By Information Hiding we mean “Showing only those details to the outside world which are necessary for the outside world and hiding all other details from the outside world.”
Real Life Examples of Information Hiding
- Your name and other personal information is stored in your brain we can’t access this information directly. For getting this information we need to ask you about it and it will be up to you how much details you would like to share with us.
- An email server may have account information of millions of people but it will share only our account information with us if we request it to send anyone else accounts information our request will be refused.
- A phone SIM card may store several phone numbers but we can’t read the numbers directly from the SIM card rather phone-set reads this information for us and if the owner of this phone has not allowed others to see the numbers saved in this phone we will not be able to see those phone numbers using phone.
In object oriented programming approach we have objects with their attributes and behaviors that are hidden from other classes, so we can say that object oriented programming follows the principle of information hiding.
In the perspective of Object Oriented Programming Information Hiding is,
“Hiding the object details (state and behavior) from the users”
Here by users we mean “an object” of another class that is calling functions of this class using the reference of this class object or it may be some other program in which we are using this class.
Information Hiding is achieved in Object Oriented Programming using the following principles,
· All information related to an object is stored within the object
· It is hidden from the outside world
· It can only be manipulated by the object itself
Advantages of Information Hiding
Following are two major advantages of information hiding. It simplifies our Object Oriented Model:
As we saw earlier that our object oriented model only had objects and their interactions hiding implementation details so it makes it easier for everyone to understand our object oriented model. It is a barrier against change propagation. As implementation of functions is limited to our class and we have only given the name of functions to user along with description of parameters so if we change implementation of function it doesn’t affect the object oriented model.
We can achieve information hiding using Encapsulation and Abstraction, so we see these two concepts in detail now.
Encapsulation
Encapsulation means “we have enclosed all the characteristics of an object in the object itself”.
Encapsulation and information hiding are much related concepts (information hiding is achieved using Encapsulation). We have seen in previous lecture that object characteristics include data members and behavior of the object in the form of functions. So we can say that Data and Behavior are tightly coupled inside an object and both the information structure and implementation details of its operations are hidden from the outer world.
(c) Explain why java is platform independent?A.1.(c)
Typically, the compiled code is the exact set of instructions the CPU requires to "execute" the program. In Java, the compiled code is an exact set of instructions for a "virtual CPU" which is required to work the same on every physical machine.
So, in a sense, the designers of the Java language decided that the language and the compiled code was going to be platform independent, but since the code eventually has to run on a physical platform, they opted to put all the platform dependent code in the JVM.
This requirement for a JVM is in contrast to your Turbo C example. With Turbo C, the compiler will produce platform dependent code, and there is no need for a JVM work-alike because the compiled Turbo C program can be executed by the CPU directly.
With Java, the CPU executes the JVM, which is platform dependent. This running JVM then executes the Java bytecode which is platform independent, provided that you have a JVM available for it to execute upon. You might say that writing Java code, you don't program for the code to be executed on the physical machine, you write the code to be executed on the Java Virtual Machine.
Q.2. (a) What are different data types in java? Explain briefly.
A.2.(a) Data types are the domains which determine what type of contents can be stored in a variable. In Java, there are two types of data types:
1. Primitive data types
2. Reference data types
Primitive Data Types
Primitive data types are non grouped pieces of data which can have only one value at a time. They are the simplest built in forms of data in Java. Other data types are made up of combination of primitive data types. Java initializes all primitive data types to default values if an initial value is not explicitly specified by the programmer. Integer and floating-point variables are initialized to 0. The char data type is initialized to null and Boolean data types are set to false.
The types byte, short, int, long, float, double, boolean and char are called primitive data types , as they are built into the Java language at low level .
The primitive data type keywords are as shown:
boolean
char
byte
short
int
long
float
double
Reference Data Types
Reference data types are made by the logical grouping of primitive data types. These are called reference data types because they contain the address of a value rather than the value itself. Arrays, objects, interfaces, enum etc. are examples of reference data types.
Integer Data Types
There are four integer data types: byte, short, int, and long. Each can handle different ranges of numbers, as shown:
Integer data type ranges
Type
|
Length
|
Minimum Value
|
Maximum Value
|
byte
|
8 bits
|
-128
|
127
|
short
|
16bits
|
-32768
|
32767
|
int
|
32 bits
|
-2,147,483,648
|
2,147,483,647
|
long
|
64bits
|
-9,223,372,036,854,775,808
|
9,223,372,036,854,775,80
|
float
|
-3.4Е+38
|
+3.4Е+38
| |
double
|
-1.7Е+308
|
+1.7Е+308
| |
boolean
|
Possible values are two - true or false
| ||
char
| |||
Object
|
null
| ||
String
|
null
|
byte: The byte data type is an 8-bit signed two's complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive).
short: The short data type is a 16-bit signed two's complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). As with
int: The int data type is a 32-bit signed two's complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive). For integral values, this data type is generally the default choice unless there is a reason (like the above) to choose something else
long: The long data type is a 64-bit signed two's complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use this data type when you need a range of values wider than those provided by int.
short: The short data type is a 16-bit signed two's complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). As with
int: The int data type is a 32-bit signed two's complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive). For integral values, this data type is generally the default choice unless there is a reason (like the above) to choose something else
long: The long data type is a 64-bit signed two's complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use this data type when you need a range of values wider than those provided by int.
char Data Types
Type char is basically a 16-bit unsigned integer that represents a Unicode value. Each printable and nonprintable character has a Unicode value. Because Java stores all characters as Unicode, it can be used with virtually any written language in the world.
Floating-Point Data Types
Type float designates that the variable is a single-precision, 32-bit, floating-point number. Type double is a double-precision, 64-bit floating-point number.
Example:
float m;
double n;
boolean Data Types
Type boolean can only have a value of true or false. Internal to Java, a Boolean value is a I-bit logical quantity. Boolean values are used in branching/looping statements to check validity of the logical expression included.
double Data Types
The double data type is a double-precision 64-bit IEEE 754 floating point. For decimal values, this data type is generally the default choice. As mentioned above, this data type should never be used for precise values, such as currency.
(b) Write a java program to create an Account class and define methods in
it, to manage saving bank account.
A.2(b)
public class SavingsAccount { private double balance; private double interest; public SavingsAccount() { balance = 0; interest = 0; } public SavingsAccount(double initialBalance, double initialInterest) { balance = initialBalance; interest = initialInterest; } public void deposit(double amount) { balance = balance + amount; } public void withdraw(double amount) { balance = balance - amount; } public void addInterest() { balance = balance + balance * interest; } public double getBalance() { return balance; } |
Q.3.(a)Explain with an example, how array of objects are created in java?
A.3.(a)
After having good command over the class and objects, you must have understood how useful the concept of class and objects can be. Now, imagine you are making a task scheduler application and you have made a 'Task' class having elements like date, time and title. Now, you have to store hundreds of tasks. And there you got the need of creating an array of objects like we had done with different data-types.
So, let's learn how to declare an array of objects.
So, let's learn how to declare an array of objects.
class Car
{
public int power;
public int Serial_no;
public Car(int p, int s)
{
power = p;
Serial_no = s;
}
}
class Ar1
{
public static void main(String[] args)
{
Car[] c;
c = new Car[10];
c[0] = new Car(800,111);
}
}
Output
Let's go through this code.
Car[] c; - This line declares that c is an array of 'Car'.
c = new Car[10] - This line will create an array 'c' of 10 elements of class 'Car'.
Car[] c; - This line declares that c is an array of 'Car'.
c = new Car[10] - This line will create an array 'c' of 10 elements of class 'Car'.
Here, only an array is created and not objects of 'Car'. To create an object, we need to use the 'new' operator with the 'Car' class.
c[0] = new Car(800,111); - This line will create an object of 'Car' on 0th element of the array 'c' and assign 800 to power and 111 to serial_no of this object.
c[0] = new Car(800,111); - This line will create an object of 'Car' on 0th element of the array 'c' and assign 800 to power and 111 to serial_no of this object.

Q.3.(b)Write a java program to demonstrate handling of multidimensional array
in java.
A.3.(b)
In Java, multidimensional arrays are actually arrays of arrays. These, as you might expect, look and act like regular multidimensional arrays. However, as you will see, there are a couple of subtle differences. To declare a multidimensional array variable, specify each additional index using another set of square brackets. For example, the following declares a two-dimensional array variable called twoD.
int twoD[][] = new int[4][5];
This allocates a 4 by 5 array and assigns it to twoD. Internally this matrix is implemented as an array of arraysof int.Conceptually, this array will look like the one shown in Figure below:
The following program numbers each element in the array from left to right, top to bottom, and then displays these values:
// Demonstrate a two-dimensional array.
class TwoDArray {
public static void main(String args[]) {
int twoD[][]= new int[4][5];
int i, j, k = 0;
for(i=0; i<4; i++)
for(j=0; j<5; j++) {
twoD[i][j] = k;
k++;
}
for(i=0; i<4; i++) {
for(j=0; j<5; j++)
System.out.print(twoD[i][j] + " ");
System.out.println();
}
}
}
This program generates the following output:
0 1 2 3 4
5 6 7 8 9
10 11 12 13 14
15 16 17 18 19
When you allocate memory for a multidimensional array, you need only specify the memory for the first (leftmost) dimension. You can allocate the remaining dimensions separately. For example, this following code allocates memory for the first dimension of twoD when it is declared. It allocates the second dimension manually.
int twoD[][] = new int[4][];
twoD[0] = new int[5];
twoD[1] = new int[5];
twoD[2] = new int[5];
twoD[3] = new int[5];
While there is no advantage to individually allocating the second dimension arrays in this situation, there may be in others. For example, when you allocate dimensions manually, you do not need to allocate the same number of elements for each dimension. As stated earlier, since multidimensional arrays are actually arrays of arrays, the length of each array is under your control. For example, the following program creates a two-dimensional array in which the sizes of the second dimension are unequal.
// Manually allocate differing size second dimensions.
class TwoDAgain {
public static void main(String args[]) {
int twoD[][] = new int[4][];
twoD[0] = new int[1];
twoD[1] = new int[2];
twoD[2] = new int[3];
twoD[3] = new int[4];
int i, j, k = 0;
for(i=0; i<4; i++)
for(j=0; j<i+1; j++) {
twoD[i][j] = k;
k++;
}
for(i=0; i<4; i++) {
for(j=0; j<i+1; j++)
System.out.print(twoD[i][j] + " ");
System.out.println();
}
}
}
This program generates the following output:
0
1 2
3 4 5
6 7 8 9
The array created by this program looks like this:

Q.3.(c) Write a java program to create Date class with proper constructor, to
create object containing date and time. Define a method to display
current date and time. Make necessary assumptions required.
A.3.(c)
Java provides the Date class available in java.util package, this class encapsulates the current date and time.
The Date class supports two constructors as shown in the following table.
Sr.No. | Constructor & Description |
---|---|
1 |
Date( )
This constructor initializes the object with the current date and time.
|
2 |
Date(long millisec)
This constructor accepts an argument that equals the number of milliseconds that have elapsed since midnight, January 1, 1970.
|
Following are the methods of the date class.
Sr.No. | Method & Description |
---|---|
1 |
boolean after(Date date)
Returns true if the invoking Date object contains a date that is later than the one specified by date, otherwise, it returns false.
|
2 |
boolean before(Date date)
Returns true if the invoking Date object contains a date that is earlier than the one specified by date, otherwise, it returns false.
|
3 |
Object clone( )
Duplicates the invoking Date object.
|
4 |
int compareTo(Date date)
Compares the value of the invoking object with that of date. Returns 0 if the values are equal. Returns a negative value if the invoking object is earlier than date. Returns a positive value if the invoking object is later than date.
|
5 |
int compareTo(Object obj)
Operates identically to compareTo(Date) if obj is of class Date. Otherwise, it throws a ClassCastException.
|
6 |
boolean equals(Object date)
Returns true if the invoking Date object contains the same time and date as the one specified by date, otherwise, it returns false.
|
7 |
long getTime( )
Returns the number of milliseconds that have elapsed since January 1, 1970.
|
8 |
int hashCode( )
Returns a hash code for the invoking object.
|
9 |
void setTime(long time)
Sets the time and date as specified by time, which represents an elapsed time in milliseconds from midnight, January 1, 1970.
|
10 |
String toString( )
Converts the invoking Date object into a string and returns the result.
|
Getting Current Date and Time
This is a very easy method to get current date and time in Java. You can use a simple Date object with toString() method to print the current date and time as follows −
Example
import java.util.Date;
public class DateDemo
{
public static void main(String args[])
{ // Instantiate a Date object Date date = new Date(); // display time and date using toString() System.out.println(date.toString());
}
}
This will produce the following result −
Output
on May 04 09:51:52 CDT 2009
Date Comparison
Following are the three ways to compare two dates −
- You can use getTime( ) to obtain the number of milliseconds that have elapsed since midnight, January 1, 1970, for both objects and then compare these two values.
- You can use the methods before( ), after( ), and equals( ). Because the 12th of the month comes before the 18th, for example, new Date(99, 2, 12).before(new Date (99, 2, 18)) returns true.
- You can use the compareTo( ) method, which is defined by the Comparable interface and implemented by Date.
Date Formatting Using SimpleDateFormat
SimpleDateFormat is a concrete class for formatting and parsing dates in a locale-sensitive manner. SimpleDateFormat allows you to start by choosing any user-defined patterns for date-time formatting.
Example
import java.util.*;
import java.text.*;
public class DateDemo
{
public static void main(String args[])
{ Date d Now = new Date( );
SimpleDateFormat ft = new SimpleDateFormat ("E yyyy.MM.dd 'at' hh:mm:ss a zzz"); System.out.println("Current Date: " + ft.format(dNow));
}
}
class Subclass-name extends Superclass-name
{
//methods and fields
}
class Employee{
float salary=40000;
}
class Programmer extends Employee{
int bonus=10000;
public static void main(String args[]){
Programmer p=new Programmer();
System.out.println("Programmer salary is:"+p.salary);
System.out.println("Bonus of Programmer is:"+p.bonus);
}
}
This will produce the following result −
Output
Current Date: Sun 2004.07.18 at 04:14:09 PM PDT
Q.4. (a) What is inheritance? How it provides flexibility in application
development? Explain with the help of an example.
A.4.(a)
Inheritance in java is a mechanism in which one object acquires all the properties and behaviors of parent object.
The idea behind inheritance in java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of parent class, and you can add new methods and fields also.
Inheritance represents the IS-A relationship, also known as parent-child relationship.
Why use inheritance in java
- For Method Overriding (so runtime polymorphism can be achieved).
- For Code Reusability.
Syntax of Java Inheritance
class Subclass-name extends Superclass-name
{
//methods and fields
}
The extends keyword indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
In the terminology of Java, a class which is inherited is called parent or super class and the new class is called child or subclass.
Java Inheritance Example
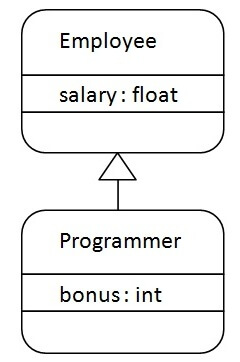
As displayed in the above figure, Programmer is the subclass and Employee is the superclass. Relationship between two classes is Programmer IS-A Employee.It means that Programmer is a type of Employee.
class Employee{
float salary=40000;
}
class Programmer extends Employee{
int bonus=10000;
public static void main(String args[]){
Programmer p=new Programmer();
System.out.println("Programmer salary is:"+p.salary);
System.out.println("Bonus of Programmer is:"+p.bonus);
}
}
(b) Explain the need of package in Java. Explain accessibility rules for
package.
Ans(b)
ackages are used in Java in order to prevent naming conflicts, to control access, to make searching/locating and usage of classes, interfaces, enumerations and annotations easier, etc.
A Package can be defined as a grouping of related types (classes, interfaces, enumerations and annotations ) providing access protection and namespace management.
Some of the existing packages in Java are −
- java.lang − bundles the fundamental classes
- java.io − classes for input , output functions are bundled in this package
Programmers can define their own packages to bundle group of classes/interfaces, etc. It is a good practice to group related classes implemented by you so that a programmer can easily determine that the classes, interfaces, enumerations, and annotations are related.
Since the package creates a new namespace there won't be any name conflicts with names in other packages. Using packages, it is easier to provide access control and it is also easier to locate the related classes.
Creating a Package
While creating a package, you should choose a name for the package and include a package statement along with that name at the top of every source file that contains the classes, interfaces, enumerations, and annotation types that you want to include in the package.
The package statement should be the first line in the source file. There can be only one package statement in each source file, and it applies to all types in the file.
If a package statement is not used then the class, interfaces, enumerations, and annotation types will be placed in the current default package.
To compile the Java programs with package statements, you have to use -d option as shown below.
javac -d Destination_folder file_name.java
Then a folder with the given package name is created in the specified destination, and the compiled class files will be placed in that folder.
Example
Let us look at an example that creates a package called animals. It is a good practice to use names of packages with lower case letters to avoid any conflicts with the names of classes and interfaces.
Following package example contains interface named animals −
/* File name : Animal.java */
package animals;
interface Animal
{
public void eat();
public void travel();
}
Now, let us implement the above interface in the same package animals −
package animals;
/* File name : MammalInt.java */
public class MammalInt implements Animal
{
public void eat()
{
System.out.println("Mammal eats");
}
public void travel()
{
System.out.println("Mammal travels");
} public int noOfLegs()
{
return 0;
}
public static void main(String args[])
{
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Now compile the java files as shown below −
$ javac -d . Animal.java
$ javac -d . MammalInt.java
(c) Explain concept of polymorphism with the help of example.
Ans(c)
Polymorphism is an object-oriented programming concept that refers to the ability of a variable, function or object to take on multiple forms. A language that features polymorphism allows developers to program in the general rather than program in the specific.
In a programming language that exhibits polymorphism, objects of classes belonging to the same hierarchical tree (inherited from a common base class) may possess functions bearing the same name, but each having different behaviors.
As an example, assume there is a base class named Animals from which the subclasses Horse, Fish and Bird are derived. Also assume that the Animals class has a function named Move, which is inherited by all subclasses mentioned. With polymorphism, each subclass may have its own way of implementing the function. So, for example, when the Move function is called in an object of the Horse class, the function might respond by displaying trotting on the screen. On the other hand, when the same function is called in an object of the Fish class, swimming might be displayed on the screen. In the case of a Bird object, it may be flying.
5. (a) What is interface? How it is different from abstract class.
A.5.(a)
An interface is a contract: The guy writing the interface says, "hey, I accept things looking that way", and the guy using the interface says "OK, the class I write looks that way".
An interface is an empty shell. There are only the signatures of the methods, which implies that the methods do not have a body. The interface can't do anything. It's just a pattern.
For example (pseudo code):
// I say all motor vehicles should look like this:
interface MotorVehicle
{
void run();
int getFuel();
}
// My team mate complies and writes vehicle looking that way
class Car implements MotorVehicle
{
int fuel;
void run()
{
print("Wrroooooooom");
}
int getFuel()
{
return this.fuel;
}
}
Implementing an interface consumes very little CPU, because it's not a class, just a bunch of names, and therefore there isn't any expensive look-up to do. It's great when it matters, such as in embedded devices.
Abstract classes
Abstract classes, unlike interfaces, are classes. They are more expensive to use, because there is a look-up to do when you inherit from them.
Abstract classes look a lot like interfaces, but they have something more: You can define a behavior for them. It's more about a guy saying, "these classes should look like that, and they have that in common, so fill in the blanks!".
For example:
// I say all motor vehicles should look like this:
abstract class MotorVehicle
{
int fuel;
// They ALL have fuel, so lets implement this for everybody.
int getFuel()
{
return this.fuel;
}
// That can be very different, force them to provide their
// own implementation.
abstract void run();
}
// My teammate complies and writes vehicle looking that way
class Car extends MotorVehicle
{
void run()
{
print("Wrroooooooom");
}
}
(b) What is an exception? Explain various causes of exceptions. Explain
different types of exceptions.
A.5.(b)
An exception (or exceptional event) is a problem that arises during the execution of a program. When an Exception occurs the normal flow of the program is disrupted and the program/Application terminates abnormally, which is not recommended, therefore, these exceptions are to be handled.
An exception can occur for many different reasons. Following are some scenarios where an exception occurs.
A user has entered an invalid data.
A file that needs to be opened cannot be found.
A network connection has been lost in the middle of communications or the JVM has run out of memory.
Some of these exceptions are caused by user error, others by programmer error, and others by physical resources that have failed in some manner.
Based on these, we have three categories of Exceptions. You need to understand them to know how exception handling works in Java.
Checked exceptions − A checked exception is an exception that occurs at the compile time, these are also called as compile time exceptions. These exceptions cannot simply be ignored at the time of compilation, the programmer should take care of (handle) these exceptions.
For example, if you use FileReader class in your program to read data from a file, if the file specified in its constructor doesn't exist, then a FileNotFoundException occurs, and the compiler prompts the programmer to handle the exception.
Example
import java.io.File;
import java.io.FileReader;
public class FilenotFound_Demo {
public static void main(String args[]) {
File file = new File("E://file.txt");
FileReader fr = new FileReader(file);
}
}
If you try to compile the above program, you will get the following exceptions.
Output
C:\>javac FilenotFound_Demo.java
FilenotFound_Demo.java:8: error: unreported exception FileNotFoundException; must be caught or declared to be thrown
FileReader fr = new FileReader(file);
Unchecked exceptions − An unchecked exception is an exception that occurs at the time of execution. These are also called as Runtime Exceptions. These include programming bugs, such as logic errors or improper use of an API. Runtime exceptions are ignored at the time of compilation.
For example, if you have declared an array of size 5 in your program, and trying to call the 6th element of the array then an ArrayIndexOutOfBoundsExceptionexception occurs.
Example
public class Unchecked_Demo {
public static void main(String args[]) {
int num[] = {1, 2, 3, 4};
System.out.println(num[5]);
}
}
If you compile and execute the above program, you will get the following exception.
Output
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 5
at Exceptions.Unchecked_Demo.main(Unchecked_Demo.java:8)
Errors − These are not exceptions at all, but problems that arise beyond the control of the user or the programmer. Errors are typically ignored in your code because you can rarely do anything about an error. For example, if a stack overflow occurs, an error will arise. They are also ignored at the time of compilation.
(c) Explain the situations in which constructors are overloaded, with the
help of example.
A.5.(c)
Constructors
Constructors are special class functions which performs initialization of every object. The Compiler calls the Constructor whenever an object is created. Constructors iitialize values to object members after storage is allocated to the object.
class A
{
int x;
public:
A(); //Constructor
};
While defining a contructor you must remeber that the name of constructor will be same as the name of the class, and contructors never have return type.
Constructors can be defined either inside the class definition or outside class definition using class name and scope resolution :: operator.
class A
{
int i;
public:
A(); //Constructor declared
};
A::A() // Constructor definition
{
i=1;
}
Types of Constructors
Constructors are of three types :
- Default Constructor
- Parametrized Constructor
- Copy COnstructor
- Default Constructor
Default constructor is the constructor which doesn't take any argument. It has no parameter.
Syntax :
class_name ()
{ Constructor Definition }
Example :
class Cube
{
int side;
public:
Cube()
{
side=10;
}
};
int main()
{
Cube c;
cout << c.side;
}
Output : 10
In this case, as soon as the object is created the constructor is called which initializes its data members.
A default constructor is so important for initialization of object members, that even if we do not define a constructor explicitly, the compiler will provide a default constructor implicitly.
class Cube
{
public:
int side;
};
int main()
{
Cube c;
cout << c.side;
}
Output : 0
In this case, default constructor provided by the compiler will be called which will initialize the object data members to default value, that will be 0 in this case.
Parameterized Constructor
These are the constructors with parameter. Using this Constructor you can provide different values to data members of different objects, by passing the appropriate values as argument.
Example :
class Cube
{
public:
int side;
Cube(int x)
{
side=x;
}
};
int main()
{
Cube c1(10);
Cube c2(20);
Cube c3(30);
cout << c1.side;
cout << c2.side;
cout << c3.side;
}
OUTPUT : 10 20 30
By using parameterized construcor in above case, we have initialized 3 objects with user defined values. We can have any number of parameters in a constructor.
Copy Constructor
These are special type of Constructors which takes an object as argument, and is used to copy values of data members of one object into other object. We will study copy constructors in detail later.
Constructor Overloading
Just like other member functions, constructors can also be overloaded. Infact when you have both default and parameterized constructors defined in your class you are having Overloaded Constructors, one with no parameter and other with parameter.
You can have any number of Constructors in a class that differ in parameter list.
class Student
{
int rollno;
string name;
public:
Student(int x)
{
rollno=x;
name="None";
}
Student(int x, string str)
{
rollno=x ;
name=str ;
}
};
int main()
{
Student A(10);
Student B(11,"Ram");
}
In above case we have defined two constructors with different parameters, hence overloading the constructors.
One more important thing, if you define any constructor explicitly, then the compiler will not provide default constructor and you will have to define it yourself.
In the above case if we write Student S; in main(), it will lead to a compile time error, because we haven't defined default constructor, and compiler will not provide its default constructor because we have defined other parameterized constructors.
6. (a) What is multithreading? Explain various applications where
multithreading may be used. Also explain how threads are created in
java.
A.6.(a)
Java is a multi-threaded programming language which means we can develop multi-threaded program using Java. A multi-threaded program contains two or more parts that can run concurrently and each part can handle a different task at the same time making optimal use of the available resources specially when your computer has multiple CPUs.
By definition, multitasking is when multiple processes share common processing resources such as a CPU. Multi-threading extends the idea of multitasking into applications where you can subdivide specific operations within a single application into individual threads. Each of the threads can run in parallel. The OS divides processing time not only among different applications, but also among each thread within an application.
Multi-threading enables you to write in a way where multiple activities can proceed concurrently in the same program.
Life Cycle of a Thread
A thread goes through various stages in its life cycle. For example, a thread is born, started, runs, and then dies. The following diagram shows the complete life cycle of a thread.
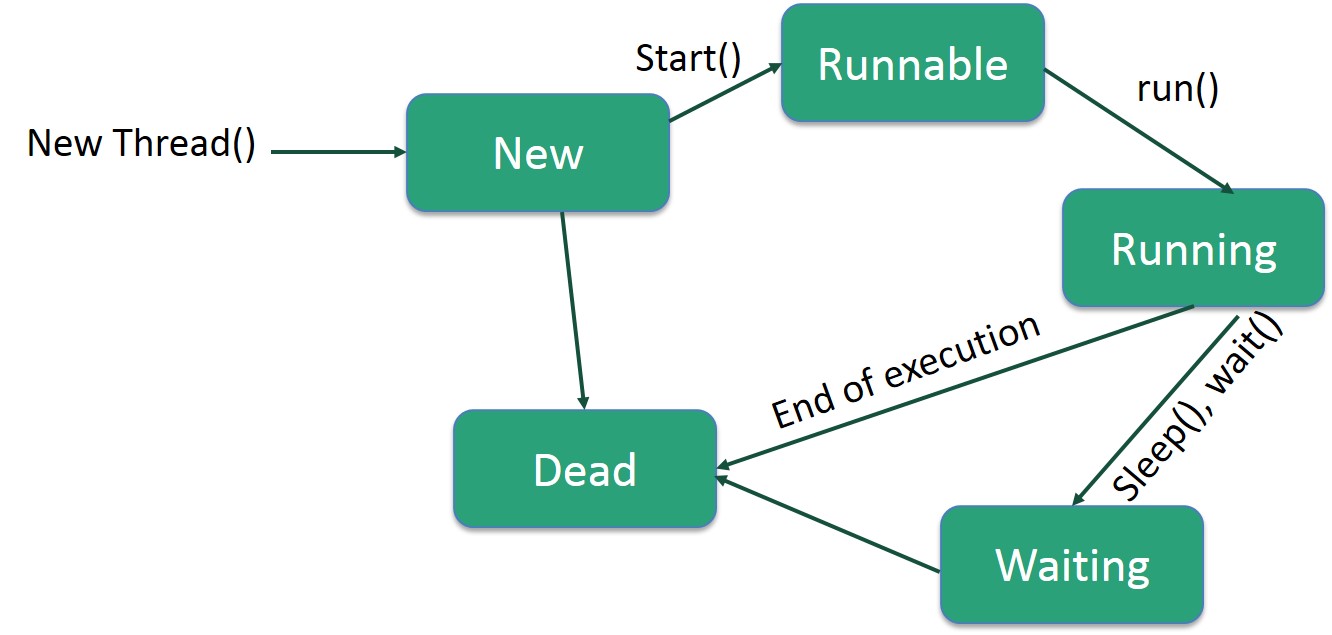
Following are the stages of the life cycle −
- New − A new thread begins its life cycle in the new state. It remains in this state until the program starts the thread. It is also referred to as a born thread.
- Runnable − After a newly born thread is started, the thread becomes runnable. A thread in this state is considered to be executing its task.
- Waiting − Sometimes, a thread transitions to the waiting state while the thread waits for another thread to perform a task. A thread transitions back to the runnable state only when another thread signals the waiting thread to continue executing.
- Timed Waiting − A runnable thread can enter the timed waiting state for a specified interval of time. A thread in this state transitions back to the runnable state when that time interval expires or when the event it is waiting for occurs.
- Terminated (Dead) − A runnable thread enters the terminated state when it completes its task or otherwise terminates.
(b) Create an Applet which take name and address of a student and convert
it into upper case.
A.6.(b)
Import java.awt.*;
import java.applet.*;
import java.awt.event.*;
/* <applet code="Stupanel" width=400 height=400>
</applet> */
public class Stupanel extends Applet implements ActionListener,ItemListener
{
String s1,s2,s3;
TextField t3,t4,t5,t6,t7;
Button tot,avg;
Checkbox c1,c2,c3,c4,m,f;
CheckboxGroup cbg;
Panel p1,p2,p3,p4;
public void init()
{
s3=" ";
tot=new Button("Total");
avg=new Button("Average");
c1=new Checkbox("MCA",true);
c2=new Checkbox("Msc Comp");
c3=new Checkbox("MSIT");
c4=new Checkbox("MSIS");
cbg=new CheckboxGroup();
m=new Checkbox("Male",cbg,false);
f=new Checkbox("Female",cbg,true);
p1=new Panel();
p1.setLayout(new GridLayout(2,2));
p1.add(new Label("Student Number "));
p1.add(new TextField(5));
p1.add(new Label("Student Name "));
p1.add(new TextField(15));
add(p1);
p2=new Panel(); p2.setLayout(new GridLayout(1,3));
p2.add(new Label("Gender"));
p2.add(m);
p2.add(f);
add(p2);
p3=new Panel(); p3.setLayout(new GridLayout(1,5));
p3.add(new Label("Degree"));
p3.add(c1); p3.add(c2); p3.add(c3); p3.add(c4);
add(p3);
p4=new Panel(); p4.setLayout(new GridLayout(6,2));
p4.add(new Label("Marks in JAVA"));
t3=new TextField(3); p4.add(t3);
p4.add(new Label("Marks in VB .Net"));
t4=new TextField(3); p4.add(t4);
p4.add(new Label("Marks In C"));
t5=new TextField(3); p4.add(t5);
p4.add(new Label("Total "));
t6=new TextField(3); p4.add(t6);
p4.add(new Label(" Average"));
t7=new TextField(3); p4.add(t7);
p4.add(tot); p4.add(avg);
tot.addActionListener(this);
avg.addActionListener(this);
c1.addItemListener(this);
c2.addItemListener(this);
c3.addItemListener(this);
c4.addItemListener(this);
m.addItemListener(this);
f.addItemListener(this);
add(p4);
}
public void paint(Graphics g)
{
int no,m1,m2,m3,tot;
float avg=0.0f;
no=m1=m2=m3=tot=0;
try
{
m1=Integer.parseInt(t3.getText());
m2=Integer.parseInt(t4.getText());
m3=Integer.parseInt(t5.getText());
}
catch(Exception e)
{
}
tot=m1+m2+m3;
avg= tot/3;
s1=String.valueOf(tot);
s2=String.valueOf(avg);
}
public boolean action(Event e,Object o)
{
repaint();
return true;
}
public void actionPerformed(ActionEvent e)
{
s3=e.getActionCommand();
if(s3.equals("Total"))
t6.setText(s1);
if(s3.equals("Average"))
t7.setText(s2);
repaint();
}
public void itemStateChanged(ItemEvent e)
{
repaint();
}
}
import java.applet.*;
import java.awt.event.*;
/* <applet code="Stupanel" width=400 height=400>
</applet> */
public class Stupanel extends Applet implements ActionListener,ItemListener
{
String s1,s2,s3;
TextField t3,t4,t5,t6,t7;
Button tot,avg;
Checkbox c1,c2,c3,c4,m,f;
CheckboxGroup cbg;
Panel p1,p2,p3,p4;
public void init()
{
s3=" ";
tot=new Button("Total");
avg=new Button("Average");
c1=new Checkbox("MCA",true);
c2=new Checkbox("Msc Comp");
c3=new Checkbox("MSIT");
c4=new Checkbox("MSIS");
cbg=new CheckboxGroup();
m=new Checkbox("Male",cbg,false);
f=new Checkbox("Female",cbg,true);
p1=new Panel();
p1.setLayout(new GridLayout(2,2));
p1.add(new Label("Student Number "));
p1.add(new TextField(5));
p1.add(new Label("Student Name "));
p1.add(new TextField(15));
add(p1);
p2=new Panel(); p2.setLayout(new GridLayout(1,3));
p2.add(new Label("Gender"));
p2.add(m);
p2.add(f);
add(p2);
p3=new Panel(); p3.setLayout(new GridLayout(1,5));
p3.add(new Label("Degree"));
p3.add(c1); p3.add(c2); p3.add(c3); p3.add(c4);
add(p3);
p4=new Panel(); p4.setLayout(new GridLayout(6,2));
p4.add(new Label("Marks in JAVA"));
t3=new TextField(3); p4.add(t3);
p4.add(new Label("Marks in VB .Net"));
t4=new TextField(3); p4.add(t4);
p4.add(new Label("Marks In C"));
t5=new TextField(3); p4.add(t5);
p4.add(new Label("Total "));
t6=new TextField(3); p4.add(t6);
p4.add(new Label(" Average"));
t7=new TextField(3); p4.add(t7);
p4.add(tot); p4.add(avg);
tot.addActionListener(this);
avg.addActionListener(this);
c1.addItemListener(this);
c2.addItemListener(this);
c3.addItemListener(this);
c4.addItemListener(this);
m.addItemListener(this);
f.addItemListener(this);
add(p4);
}
public void paint(Graphics g)
{
int no,m1,m2,m3,tot;
float avg=0.0f;
no=m1=m2=m3=tot=0;
try
{
m1=Integer.parseInt(t3.getText());
m2=Integer.parseInt(t4.getText());
m3=Integer.parseInt(t5.getText());
}
catch(Exception e)
{
}
tot=m1+m2+m3;
avg= tot/3;
s1=String.valueOf(tot);
s2=String.valueOf(avg);
}
public boolean action(Event e,Object o)
{
repaint();
return true;
}
public void actionPerformed(ActionEvent e)
{
s3=e.getActionCommand();
if(s3.equals("Total"))
t6.setText(s1);
if(s3.equals("Average"))
t7.setText(s2);
repaint();
}
public void itemStateChanged(ItemEvent e)
{
repaint();
}
}

7.(a) What is object serialization? Explain working of object serialization.
A.7.(a)
Java provides a mechanism, called object serialization where an object can be represented as a sequence of bytes that includes the object's data as well as information about the object's type and the types of data stored in the object.
After a serialized object has been written into a file, it can be read from the file and deserialized that is, the type information and bytes that represent the object and its data can be used to recreate the object in memory.
Most impressive is that the entire process is JVM independent, meaning an object can be serialized on one platform and deserialized on an entirely different platform.
Classes ObjectInputStream and ObjectOutputStream are high-level streams that contain the methods for serializing and deserializing an object.
The ObjectOutputStream class contains many write methods for writing various data types, but one method in particular stands out −
public final void writeObject(Object x) throws IOException
The above method serializes an Object and sends it to the output stream. Similarly, the ObjectInputStream class contains the following method for deserializing an object −
public final Object readObject() throws IOException, ClassNotFoundException
This method retrieves the next Object out of the stream and deserializes it. The return value is Object, so you will need to cast it to its appropriate data type.
To demonstrate how serialization works in Java, I am going to use the Employee class that we discussed early on in the book. Suppose that we have the following Employee class, which implements the Serializable interface −
Example
public class Employee implements java.io.Serializable
{
public String name;
public String address;
public transient int SSN;
public int number;
public void mailCheck()
{
System.out.println("Mailing a check to " + name + " " + address);
}
}
- The class must implement the java.io.Serializable interface.
- All of the fields in the class must be serializable. If a field is not serializable, it must be marked transient.
If you are curious to know if a Java Standard Class is serializable or not, check the documentation for the class. The test is simple: If the class implements java.io.Serializable, then it is serializable; otherwise, it's not.
Serializing an Object
The ObjectOutputStream class is used to serialize an Object. The following SerializeDemo program instantiates an Employee object and serializes it to a file.
When the program is done executing, a file named employee.ser is created. The program does not generate any output, but study the code and try to determine what the program is doing.
Note − When serializing an object to a file, the standard convention in Java is to give the file a .ser extension.
Example
import java.io.*;
public class SerializeDemo
{
public static void main(String [] args)
{
Employee e = new Employee();
e.name = "Reyan Ali";
e.address = "Phokka Kuan, Ambehta Peer";
e.SSN = 11122333; e.number = 101;
try
{
FileOutputStream fileOut = new FileOutputStream("/tmp/employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(e);
out.close();
fileOut.close();
System.out.printf("Serialized data is saved in /tmp/employee.ser");
}
catch(IOException i)
{ i.printStackTrace();
}
}
}
(b) Explain different stream classes in java.
A.7.(b)
Stream Classes
In Java, a stream is a path along which the data flows. Every stream has a source and a destination. We can build a complex file processing sequence using a series of simple stream operations. Two fundamental types of streams are Writing streams and Reading streams. While an Writing streams writes data into a source(file) , an Reading streamsis used to read data from a source(file).
(c) Explain StringBuffer class and its various methods.
A.7.(c)
The java.lang.StringBuffer class is a thread-safe, mutable sequence of characters. Following are the important points about StringBuffer −
- A string buffer is like a String, but can be modified.
- It contains some particular sequence of characters, but the length and content of the sequence can be changed through certain method calls.
- They are safe for use by multiple threads.
- Every string buffer has a capacity.
Class Declaration
Following is the declaration for java.lang.StringBuffer class −
public final class StringBuffer
extends Object
implements Serializable, CharSequence
Class constructors
S.N. | Constructor & Description |
---|---|
1 |
StringBuffer()
This constructs a string buffer with no characters in it and an initial capacity of 16 characters.
|
2 |
StringBuffer(CharSequence seq)
This constructs a string buffer that contains the same characters as the specified CharSequence.
|
3 |
StringBuffer(int capacity)
This constructs a string buffer with no characters in it and the specified initial capacity.
|
4 |
StringBuffer(String str)
This constructs a string buffer initialized to the contents of the specified string.
|
8. (a) What is proxy server? Explain URL class and its methods in java.
A.8.(a)
The Java URL class represents an URL. URL is an acronym for Uniform Resource Locator. It points to a resource on the World Wide Web. For example:
http://www.javatpoint.com/java-tutorial
A URL contains many information:
Protocol: In this case, http is the protocol.
Server name or IP Address: In this case, www.javatpoint.com is the server name.
Port Number: It is an optional attribute. If we write http//ww.javatpoint.com:80/sonoojaiswal/ , 80 is the port number. If port number is not mentioned in the URL, it returns -1.
File Name or directory name: In this case, index.jsp is the file name.
Commonly used methods of Java URL class
The java.net.URL class provides many methods. The important methods of URL class are given below.
Method Description
public String getProtocol() it returns the protocol of the URL.
public String getHost() it returns the host name of the URL.
public String getPort() it returns the Port Number of the URL.
public String getFile() it returns the file name of the URL.
public URLConnection openConnection() it returns the instance of URLConnection i.e. associated with this URL.
Example of Java URL class
//URLDemo.java
import java.io.*;
import java.net.*;
public class URLDemo{
public static void main(String[] args){
try{
URL url=new URL("http://www.javatpoint.com/java-tutorial");
System.out.println("Protocol: "+url.getProtocol());
System.out.println("Host Name: "+url.getHost());
System.out.println("Port Number: "+url.getPort());
System.out.println("File Name: "+url.getFile());
}catch(Exception e){System.out.println(e);}
}
}
Output:
Protocol: http
Host Name: www.javatpoint.com
Port Number: -1
(b) Explain sending UDP Datagrams with the help of an example.
A.8.(b)
Java DatagramSocket class represents a connection-less socket for sending and receiving datagram packets.
A datagram is basically an information but there is no guarantee of its content, arrival or arrival time.
Commonly used Constructors of DatagramSocket class.
DatagramSocket() throws SocketEeption: it creates a datagram socket and binds it with the available Port Number on the localhost machine.
DatagramSocket(int port) throws SocketEeption: it creates a datagram socket and binds it with the given Port Number.
DatagramSocket(int port, InetAddress address) throws SocketEeption: it creates a datagram socket and binds it with the specified port number and host address.
Java DatagramPacket class
Java DatagramPacket is a message that can be sent or received. If you send multiple packet, it may arrive in any order. Additionally, packet delivery is not guaranteed.
Commonly used Constructors of DatagramPacket class.
DatagramPacket(byte[] barr, int length): it creates a datagram packet. This constructor is used to receive the packets.
DatagramPacket(byte[] barr, int length, InetAddress address, int port): it creates a datagram packet. This constructor is used to send the packets.
Example of Sending DatagramPacket by DatagramSocket
//DSender.java
import java.net.*;
public class DSender{
public static void main(String[] args) throws Exception {
DatagramSocket ds = new DatagramSocket();
String str = "Welcome java";
InetAddress ip = InetAddress.getByName("127.0.0.1");
DatagramPacket dp = new DatagramPacket(str.getBytes(), str.length(), ip, 3000);
ds.send(dp);
ds.close();
}
}
Example of Receiving DatagramPacket by DatagramSocket
//DReceiver.java
import java.net.*;
public class DReceiver{
public static void main(String[] args) throws Exception {
DatagramSocket ds = new DatagramSocket(3000);
byte[] buf = new byte[1024];
DatagramPacket dp = new DatagramPacket(buf, 1024);
ds.receive(dp);
String str = new String(dp.getData(), 0, dp.getLength());
System.out.println(str);
ds.close();
}
}
(c) What is servlet ? Explain GET and POST methods of servlet.
A.8.(c)
GET Method
The GET method sends the encoded user information appended to the page request. The page and the encoded information are separated by the ? (question mark) symbol as follows :
http://www.test.com/hello?key1 = value1&key2 = value2
The GET method is the default method to pass information from browser to web server and it produces a long string that appears in your browser's Location:box. Never use the GET method if you have password or other sensitive information to pass to the server. The GET method has size limitation: only 1024 characters can be used in a request string.
This information is passed using QUERY_STRING header and will be accessible through QUERY_STRING environment variable and Servlet handles this type of requests using doGet() method.
POST Method
A generally more reliable method of passing information to a backend program is the POST method. This packages the information in exactly the same way as GET method, but instead of sending it as a text string after a ? (question mark) in the URL it sends it as a separate message. This message comes to the backend program in the form of the standard input which you can parse and use for your processing. Servlet handles this type of requests using doPost() method.
Reading Form Data using Servlet
Servlets handles form data parsing automatically using the following methods depending on the situation −
getParameter() − You call request.getParameter() method to get the value of a form parameter.
getParameterValues() − Call this method if the parameter appears more than once and returns multiple values, for example checkbox.
getParameterNames() − Call this method if you want a complete list of all parameters in the current request.
GET Method Example using URL
Here is a simple URL which will pass two values to HelloForm program using GET method.
http://localhost:8080/HelloForm?first_name = ZARA&last_name = ALI
Given below is the HelloForm.java servlet program to handle input given by web browser. We are going to use getParameter() method which makes it very easy to access passed information −
// Import required java libraries
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
// Extend HttpServlet class
public class HelloForm extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Set response content type
response.setContentType("text/html");
PrintWriter out = response.getWriter();
String title = "Using GET Method to Read Form Data";
String docType =
"<!doctype html public \"-//w3c//dtd html 4.0 " + "transitional//en\">\n";
out.println(docType +
"<html>\n" +
"<head><title>" + title + "</title></head>\n" +
"<body bgcolor = \"#f0f0f0\">\n" +
"<h1 align = \"center\">" + title + "</h1>\n" +
"<ul>\n" +
" <li><b>First Name</b>: "
+ request.getParameter("first_name") + "\n" +
" <li><b>Last Name</b>: "
+ request.getParameter("last_name") + "\n" +
"</ul>\n" +
"</body>
</html>"
);
}
}
Please upload MCSL 25
ReplyDeleteThis comment has been removed by a blog administrator.
ReplyDelete