Q.1.(a)
A.1.
#include<iostream.h>
#include<conio.h>
float average_marks(float []);
float highest_marks(float []);
float lowest_marks(float []);
main()
{
clrscr();
float marks[10]={0};
cout<<"\n Enter the marks of the ten students : "<<endl;
cout<<"\n\t Student No. Marks Obtained"<<endl;
for(int count_1=0;count_1<10;count_1++)
{
cout<<"\t\t"<<count_1+1<<"\t\t";
cin>>marks[count_1];
}
getch();
clrscr();
cout<<"\n ******************** Result Sheet ****************"<<endl;
cout<<"\n Total Marks = 100"<<endl;
cout<<" Average Marks = "<<average_marks(marks)<<endl;
cout<<" Highest Marks obtained = "<<highest_marks(marks)<<endl;
cout<<" Lowest Marks obtained = "<<lowest_marks(marks)<<endl;
cout<<"\n Student No. Marks Obtained Diff. from Average Marks Status"<<endl;
int y=10;
for(int count_2=0;count_2<10;count_2++)
{
cout<<"\t"<<count_2+1<<"\t\t"<<marks[count_2];
gotoxy(42,y);
cout<<marks[count_2]-average_marks(marks)<<"\t\t "<<
((marks[count_2]>=50)?"Pass":"Fail")<<endl;
y++;
}
getch();
return 0;
}
/*************************************************************************///------------------------ average_marks(float []) --------------------///*************************************************************************/float average_marks(float marks[])
{
float sum=0;
for(int count=0;count<10;count++)
sum+=marks[count];
return sum/10;
}
/*************************************************************************///--------------------- highest_marks(float []) -----------------------///*************************************************************************/float highest_marks(float marks[])
{
float highest=marks[0];
for(int count=0;count<10;count++)
{
if(marks[count]>highest)
highest=marks[count];
}
return highest;
}
/*************************************************************************///---------------------- lowest_marks(float []) -----------------------///*************************************************************************/float lowest_marks(float marks[])
{
float lowest=marks[0];
for(int count=0;count<10;count++)
{
if(marks[count]<lowest)
lowest=marks[count];
}
return lowest;
}
Q.1.(b)
A.1.(b)
#include<iostream>
using namespace std;
int area(int);
int area(int,int);
float area(float);
float area(float,float);
int main()
{
int s,l,b;
float r,bs,ht;
cout<<"Enter side of a square:";
cin>>s;
cout<<"Enter length and breadth of rectangle:";
cin>>l>>b;
cout<<"Enter radius of circle:";
cin>>r;
cout<<"Enter base and height of triangle:";
cin>>bs>>ht;
cout<<"Area of square is"<<area(s);
cout<<"\nArea of rectangle is "<<area(l,b);
cout<<"\nArea of circle is "<<area(r);
cout<<"\nArea of triangle is "<<area(bs,ht);
}
int area(int s)
{
return(s*s);
}
int area(int l,int b)
{
return(l*b);
}
float area(float r)
{
return(3.14*r*r);
}
float area(float bs,float ht)
{
return((bs*ht)/2);
}
Sample InputEnter side of a square:2
Enter length and breadth of rectangle:3 6
Enter radius of circle:3
Enter base and height of triangle:4 4
Sample Output
Area of square is4
Area of rectangle is 18
Area of circle is 28.26
Area of triangle is 8
Enter length and breadth of rectangle:3 6
Enter radius of circle:3
Enter base and height of triangle:4 4
Sample Output
Area of square is4
Area of rectangle is 18
Area of circle is 28.26
Area of triangle is 8
Q.2.(a)
A.2.(a)
An exception is a problem that arises during the execution of a program. A C++ exception is a response to an exceptional circumstance that arises while a program is running, such as an attempt to divide by zero.
Exceptions provide a way to transfer control from one part of a program to another. C++ exception handling is built upon three keywords: try, catch, and throw.
- throw: A program throws an exception when a problem shows up. This is done using a throw keyword.
- catch: A program catches an exception with an exception handler at the place in a program where you want to handle the problem. The catch keyword indicates the catching of an exception.
- try: A try block identifies a block of code for which particular exceptions will be activated. It's followed by one or more catch blocks.
Assuming a block will raise an exception, a method catches an exception using a combination of the try and catch keywords. A try/catch block is placed around the code that might generate an exception.
#include<iostream.h>
#include<conio.h>
#include<process.h>
#include<exception.h>
#include<conio.h>
#include<process.h>
#include<exception.h>
void matsum()
{
int m, n, i, j, first[10][10], second[10][10], sum[10][10];
try { cout << “Enter the number of rows and columns of matrix “;
cin >> m >> n;
cout << “Enter the elements of first matrix\n”;
if (m>10 || n>10) //exit(0);
throw 1;
}
catch(int)
{ cout<<“subscript invalid”;
}
for ( i = 0 ; i < m ; i++ )
for ( j = 0 ; j < n ; j++ )
cin >> first[i][j];
cout << “Enter the elements of second matrix\n”;
for ( i = 0 ; i < m ;i++ )
for ( j = 0 ; j < n ; j++ )
cin >> second[i][j];
for ( i = 0 ; i < m ; i++ )
for ( j = 0 ; j < n ; j++ )
sum[i][j] = first[i][j] + second[i][j];
cout << “Sum of entered matrices:-\n”;
for ( i = 0 ; i < m ; i++ )
{
for ( j = 0 ; j < n ; j++ )
cout << sum[i][j] << “\t”;
cout << endl;
}
}
{
int m, n, i, j, first[10][10], second[10][10], sum[10][10];
try { cout << “Enter the number of rows and columns of matrix “;
cin >> m >> n;
cout << “Enter the elements of first matrix\n”;
if (m>10 || n>10) //exit(0);
throw 1;
}
catch(int)
{ cout<<“subscript invalid”;
}
for ( i = 0 ; i < m ; i++ )
for ( j = 0 ; j < n ; j++ )
cin >> first[i][j];
cout << “Enter the elements of second matrix\n”;
for ( i = 0 ; i < m ;i++ )
for ( j = 0 ; j < n ; j++ )
cin >> second[i][j];
for ( i = 0 ; i < m ; i++ )
for ( j = 0 ; j < n ; j++ )
sum[i][j] = first[i][j] + second[i][j];
cout << “Sum of entered matrices:-\n”;
for ( i = 0 ; i < m ; i++ )
{
for ( j = 0 ; j < n ; j++ )
cout << sum[i][j] << “\t”;
cout << endl;
}
}
void main()
{
matsum();
getch();
{
matsum();
getch();
}
Q.2.(b)A.2.(b)
#include<iostream.h>#include<conio.h>#include<string.h>#include<fstream.h>#include<stdlib.h>void main(){ clrscr(); ifstream ifile; char s[100], fname[20]; cout<<"Enter file name to read and display its content (like file.txt) : "; cin>>fname; ifile.open(fname); if(!ifile) { cout<<"Error in opening file..!!"; getch(); exit(0); } while(ifile.eof()==0) { ifile>>s; cout<<s<<" "; } cout<<"\n"; ifile.close(); getch();}
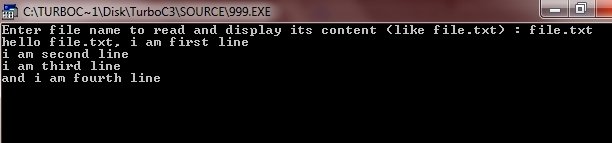
No comments:
Post a Comment